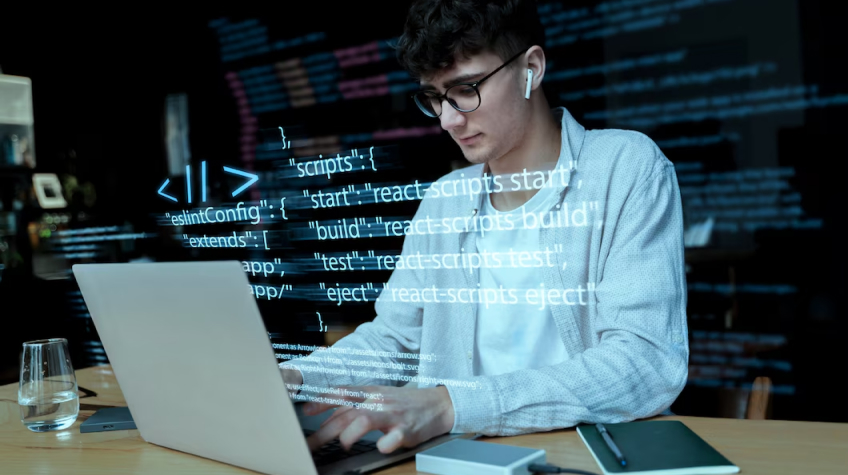
APIs serve as programmatic solutions for app interfaces, as well as making up the backbones of modern systems that enable communication among applications, thus making them connect. API testing 🧪 is the center through which they can be sure that the APIs operate well so that developers can program the application according to the specification..📋
A test script in API testing is a structured series of steps used to validate that the API performs reliably across different scenarios. No matter what you are testing for, be it proper response, ⚠️ error handling and performance! writing a good test script means your API is unbreakable and easy to be integrated to your system.
This guide will take you step by step through how to Write test script for API testing 🧑💻, including everything from the setup to execution, thus you will be able to validate your APIs with confidence.
Table of Content
- How to Write a Test Script for API Testing: A Complete Guide
What is API Testing?
API testing is a type of software testing that focuses on verifying the functionality, reliability, performance, and security of APIs (Application Programming Interfaces). It ensures that APIs return the correct responses for valid requests, handle invalid inputs gracefully, and meet performance benchmarks under various conditions. Unlike UI testing, API testing operates at the business logic layer, directly validating the communication between software systems without relying on the application’s user interface.
➢ Key objectives:
- Ensure the API returns the correct response for valid requests.
- Verify it handles invalid or edge-case requests gracefully.
- Test for performance bottlenecks and vulnerabilities.
Types of API Testing
- Functional Testing: Validates API functionality against defined requirements.
- Performance Testing: Measures response times, throughput, and scalability.
- Security Testing: Ensures APIs are secure against vulnerabilities and attacks.
- Load Testing: Tests the API under heavy traffic conditions.
- Reliability Testing: Ensures consistent performance over time.
- Negative Testing: Validates API responses to invalid requests.
Tools for API Testing
- Postman: Widely used for manual and automated API testing.
- SoapUI: Great for SOAP and REST API testing.
- JMeter: Excellent for performance testing.
- Swagger: API documentation tool with testing capabilities.
- RestAssured: A Java library for automated REST API testing.
- Katalon Studio: Provides an easy-to-use interface for API and web testing.
Preparing for API Test Script Writing
Before diving into test script writing, preparation is key.
➢ Gather API Documentation
- Endpoint URLs: Base URLs and specific endpoints.
- Request Methods: GET, POST, PUT, DELETE, etc.
- Parameters: Headers, query parameters, and body payloads.
- Response Codes: Expected status codes like 200, 400, 404, 500.
- Authentication: Details about API keys, OAuth tokens, or other mechanisms.
➢ Define Test Scenarios
- Positive scenarios (e.g., valid inputs).
- Negative scenarios (e.g., invalid inputs).
- Boundary conditions.
➢ Set Up Your Environment
- Install the required tools (e.g., Postman, RestAssured).
- Configure the environment (e.g., staging or production).
- Ensure you have the necessary access rights.
Step-by-Step Guide to Write a Test Script for API Testing
Step 1: Understand the API
Before starting, gain a thorough understanding of the API. This step is foundational, as your test script’s quality depends on how well you know the API’s behavior.
➢ Key Actions:
- Review API Documentation:
- Understand the endpoints, request methods (GET, POST, PUT, DELETE), required parameters, and response formats.
- Example: A GET /users endpoint might require a query parameter like user_id.
- Study Response Codes: Familiarize yourself with expected HTTP status codes for various requests.
- 200 OK for successful requests.
- 400 Bad Request for invalid inputs.
- 401 Unauthorized for missing/invalid authentication.
- Identify Authentication Requirements:
- Does the API use API keys, OAuth tokens, or session-based authentication?
➢ Output of Step 1:
- A checklist of endpoints, parameters, headers, and expected responses.
- A clear understanding of how the API is supposed to function.
Step 2: Set Up the Tool
Choose and configure the testing tool. Tools like Postman, RestAssured, or SoapUI make it easier to organize test cases and automate scripts.
➢ Key Differences Between Tools:
- Postman: Ideal for beginners and manual testing with an intuitive interface.
- RestAssured: A Java-based library suitable for automated testing.
- SoapUI: Perfect for SOAP and REST APIs, offering advanced mocking and load testing capabilities.
➢ Steps to Set Up (Example with Postman):
- Install Postman: Download and install the tool from Postman.
- Create a New Collection:
- Group related API tests for better organization.
- Example: A “User Management” collection might include tests for creating, fetching, updating, and deleting users.
- Configure Environment Variables: Use variables like {{base_url}} or {{auth_token}} to make tests reusable across environments (e.g., development, staging, production).
Step 3: Write the Test Cases
Clearly outline the test cases you want to execute. Each test case should specify:
- Test Objective: What are you testing? (e.g., Verify the API returns the correct response for valid user ID.)
- Request Details: HTTP method, endpoint, headers, parameters, and payload.
- Expected Outcome: Response status code, body content, and headers.
➢ Example Test Case Table:
Test Case | Request | Input/Parameters | Expected Response |
---|---|---|---|
Fetch valid user details | GET /users | user_id=123 | 200 OK with user details in JSON |
Fetch invalid user details | GET /users | user_id=999 | 404 Not Found |
Create a new user | POST /users | JSON payload with user data | 201 Created with user ID |
➢ Test Types to Include:
- Positive Tests: Valid inputs return expected results.
- Negative Tests: Invalid inputs trigger appropriate error handling.
- Boundary Tests: Test edge cases (e.g., maximum payload size).
- Security Test Cases: Test for authentication and authorization issues, such as invalid tokens or missing headers.
- Performance Test Cases: Assess the response time and throughput of the API.
Step 4: Write the Script
Create the test script using your chosen tool. Depending on the tool, scripting can range from simple GUI-based configurations to code-level scripting.
➢ Using Postman:
- Pre-request Scripts: Configure setup tasks (e.g., setting authentication tokens).
- Test Scripts: Use JavaScript to validate responses.
➢ Postman Example (JavaScript):
// Test for 200 status code
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
// Test for specific property in the response
pm.test("Response has 'user_id'", function () {
let jsonData = pm.response.json();
pm.expect(jsonData).to.have.property("user_id");
});
// Validate response time
pm.test("Response time is under 300ms", function () {
pm.expect(pm.response.responseTime).to.be.below(300);
});
➢ Using RestAssured (Java):
import io.restassured.RestAssured;
import io.restassured.response.Response;
public class ApiTest {
public static void main(String[] args) {
RestAssured.baseURI = "https://api.example.com";
Response response = RestAssured
.given()
.header("Authorization", "Bearer <token>")
.when()
.get("/users/123");
// Assertions
assert response.getStatusCode() == 200;
assert response.jsonPath().get("user_id") != null;
}
}
➢ Using Python (Requests Library):
Python’s Requests library offers simplicity:
import requests
url = "https://api.example.com/users"
headers = {"Authorization": "Bearer <token>"}
response = requests.get(url, headers=headers)
# Assertions
assert response.status_code == 200, "Expected status code 200"
assert "user_id" in response.json(), "Response should contain user_id"
Step 5: Run the Test
Execute the test script and evaluate the results.
➢ For Manual Tests (Postman):
- Click Send to execute the request.
- Check the response body, status code, and headers.
- Validate against expected outcomes.
➢ For Automated Tests (RestAssured or Newman):
- Execute the script using an IDE or command-line tool.
- Analyze results in the console or test report.
➢ Running Postman Tests with Newman (Bash):
newman run collection.json -e environment.json
Step 6: Log and Report Results
Document the test outcomes for tracking and debugging purposes.
➢ Tools for Reporting:
- Postman: Offers built-in reporting for manual tests.
- Newman: Use with Jenkins or GitHub Actions for CI/CD pipelines.
- Custom Reporting: Write logs in code for more control.
➢ Report Checklist:
- Status of each test case (Pass/Fail).
- Details of failed tests (error codes, mismatched responses).
- Execution time and performance metrics.
Step 7: Debug and Refine
If tests fail, debug the issues:
- Inspect Request: Verify headers, parameters, and payload.
- Check API Logs: Collaborate with developers to analyze server-side logs.
- Refactor Script: Update assertions or inputs to align with API changes.
Also Read: How to Use AI in Software Testing
Best Practices for API Testing
- Start with Functional Tests: Ensure basic functionality works before diving into performance or security tests.
- Use Automation: Automate repetitive tests to save time and improve coverage.
- Validate All Response Elements: Test headers, status codes, and body content.
- Leverage Mock Servers: Use mock servers for testing during early development.
- Incorporate Negative Tests: Test how the API handles invalid requests.
- Monitor API Performance: Regularly test for performance under different conditions.
Automating API Testing
Automation can save time and ensure consistency. Here’s how to automate API testing:
- Choose an Automation Framework: Tools like RestAssured, Postman’s Newman, or JMeter are great options.
- Integrate with CI/CD Pipelines: Incorporate automated tests into Jenkins, GitHub Actions, or other CI/CD tools.
- Write Maintainable Tests: Use modular and reusable test scripts.
➢ Example using Postman + Newman (Bash):
newman run your-collection.json -e your-environment.json
Common Challenges in API Testing and How to Overcome Them
- Lack of Documentation: Collaborate with developers to fill gaps.
- Dynamic Data: Use dynamic variables in tests (e.g., timestamps).
- Authentication Issues: Ensure proper setup for API keys or tokens.
- Unstable APIs: Coordinate with development teams to stabilize the API.
Also Read: Debugging Techniques in Software Testing
Conclusion
API testing is a basic step in ensuring the quality and security of software. To effectively write test script for API testing, Beginers need to follow a structured approach and the right tools. Go for tools like Postman and RestAssured and start practicing simple test cases to build your foundational skills. slowly improve the efficiency and reusability of your test scripts, adapting them to meet the developing requirements of your APIs. Including API testing in your software development process guarantees that you build robust, secured, and well-performing APIs.
Thanks for Reading! 🪲