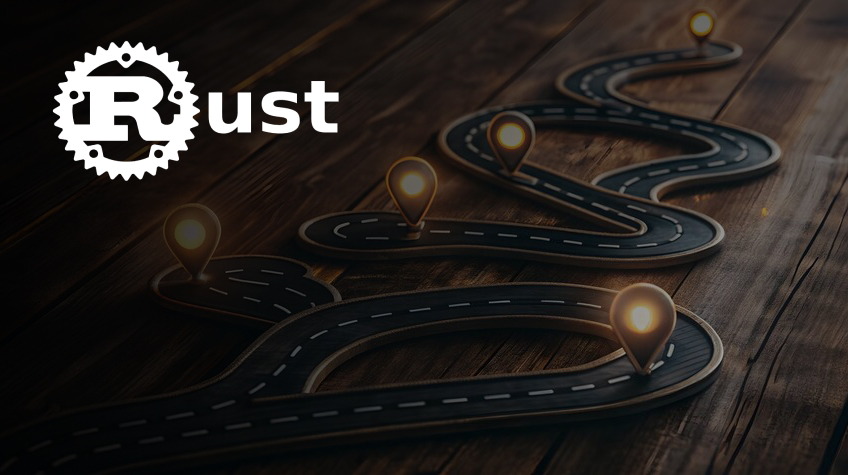
IT development industry is the most dynamic field which requires one to always be one step ahead of others if they have aspirations to develop a career in this field. As we move towards 2025, Rust becomes one of the successful and future-proof programming language that is known for its focus on safety, concurrency and performance. In this article, let’s find out about Rust Developer Roadmap and what you need to know before you become a Rust expert in 2025.
No matter if you are a newcomer who is just stepping up to the tech field or if you are an experienced developer who wants to master Rust, understanding the way ahead will help you prepare properly.
Table of Content
- How to Become a Rust Developer in 2025
This roadmap is not just for learning Rust but also for making you curious to exploit its extraordinary features to solve real-world issues and thus grow personally and in the tech industry at large. Let’s begin our path of being a Rust developer in 2025.
Why Rust Programming Language is Popular?
Rust is popular for combining high performance with memory safety, achieved without a garbage collector. Its unique ownership model prevents common bugs, such as null pointer dereferencing and data races, making Rust both fast and reliable for systems-level programming.
Additionally, Rust’s concurrency model, rich tooling, and expanding ecosystem have driven its adoption across various fields, from web development to embedded systems. These features, along with an active and supportive community, make Rust a favorite among developers looking for a language that’s as safe as it is powerful.
- Memory Safety: Ensures memory safety at compile-time using ownership, borrowing, and lifetimes, preventing common bugs.
- No Garbage Collection: Achieves high performance without a garbage collector, making it ideal for low-latency and high-performance applications.
- Concurrency: Rust’s concurrency model enables safe, efficient multi-threading, reducing the risk of data races.
- Versatile: Used across domains like system programming, web development, blockchain, and game development.
- Growing Ecosystem: A rich ecosystem with libraries and frameworks (e.g., Actix, Rocket, Bevy) supports various types of development.
- Supportive Community: A collaborative, active community and excellent documentation make learning Rust approachable.
- Backed by Industry: Supported by companies like Mozilla, Microsoft, and Amazon, increasing its relevance and job opportunities.
Rust Career Pathways
- Systems Programming: Build operating systems, networking, and embedded software.
- Web Development: Develop secure, high-performance backend services.
- Blockchain: Create decentralized applications, especially for platforms like Solana.
- Game Development: Use Rust game engines and performance-critical components for Game Development.
- Embedded Systems: Program IoT and microcontrollers with memory safety.
- DevOps Tools: Build fast, reliable CLI tools and system utilities.
- Data Processing: Implement high-speed data pipelines and ML tasks.
- Cybersecurity: Develop secure network services and security tools.
- Edge AI: Use Rust for low-latency, on-device AI and edge computing.
Rust Developer Roadmap
1. Learn the Fundamentals of Programming (If Needed)
If you’re new to programming, start by mastering core concepts in any beginner-friendly language (e.g., Python or JavaScript) to establish a foundation. Aim to understand:
- Data Types (strings, integers, booleans)
- Control Flow (conditionals and loops)
- Basic Data Structures (arrays, lists, and maps)
- Functions and Error Handling
- Object-Oriented and Functional Programming Basics
Suggested Learning Path: Use free resources like Codecademy, freeCodeCamp, or MDN Web Docs to build a foundational understanding of programming logic and syntax.
2. Setting Up the Rust Development Environment
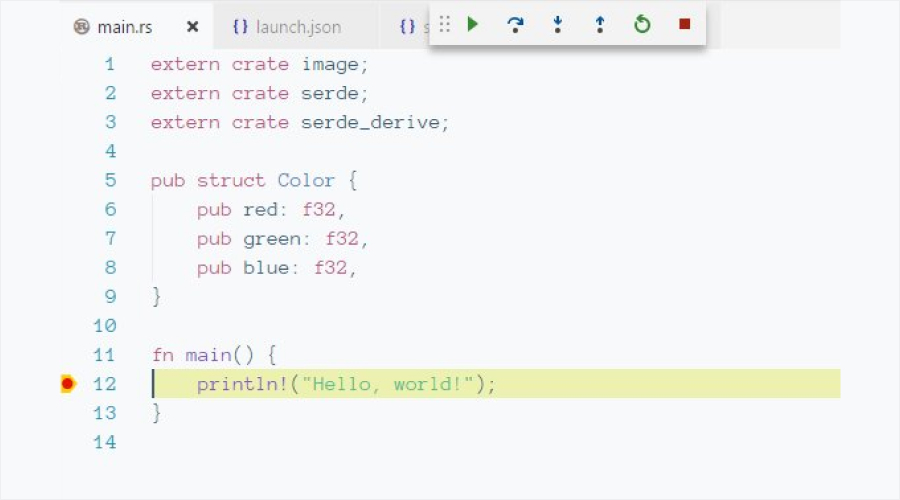
To work with Rust Programming Language, you’ll need to set up a development environment. Follow these steps:
- Install Rust: Start by installing the Rust toolchain through Rustup. This installation includes the compiler (rustc) and package manager (cargo).
- Choose an IDE: Visual Studio Code, IntelliJ IDEA (with Rust plugin), and CLion are popular IDEs for Rust development. Install extensions for Rust, like rust-analyzer, to benefit from auto-completion, error highlighting, and refactoring tools.
- Use Rust Tooling:
- Cargo: Rust’s build and package manager, essential for managing dependencies, building projects, and running tests.
- Clippy: A linter that offers suggestions for idiomatic Rust code.
- Rustfmt: A formatting tool that ensures your code adheres to Rust’s style guidelines.
Suggested Learning Path: Follow the Rust Book setup guide.
3. Master Rust Language Basics
Start with the language fundamentals, focusing on Rust’s unique syntax and structure.
- Basic Syntax and Concepts: Understand Rust’s variables, functions, and control flow. Rust’s variables are immutable by default (use let mut for mutable).
- Data Types and Structs: You will work with Rust’s primitive data types, tuples, arrays and more complex data structures like structs (user-defined types). These are the basic fundamentals that you should know.
- Ownership and Borrowing: One of Rust’s defining features is its ownership model. Learn how Rust’s ownership, borrowing, and references work to manage memory safely.
- Control Flow: Learn conditionals, loops, and pattern matching (match and if let), which add power and flexibility to control flow in Rust.
Suggested Learning Path: Progress through the Rust Book’s chapters, starting with Understanding Ownership, which is crucial to writing Rust effectively.
4. Delving Into Ownership, Borrowing, and Lifetimes
Rust’s memory safety is ensured through its strict rules around ownership, borrowing, and lifetimes. These concepts are challenging but foundational.
- Ownership Rules: Every value in Rust has a single owner. When the owner goes out of scope, the value is automatically cleaned up.
- Borrowing and References: Borrowing enables passing references to data without transferring ownership.
- Lifetimes: Use lifetimes to ensure references are valid and do not outlive their data.
Mastering these concepts is crucial for building efficient, error-free Rust applications. Practice reading and analyzing code to understand how ownership works in complex scenarios.
Suggested Learning Path: Complete Rustlings exercises focused on ownership, borrowing, and lifetimes.
5. Understanding Structs, Enums, and Pattern Matching
- Structs: Define custom data types that can bundle multiple values, helping organize complex data.
- Enums: Enums allow a value to represent multiple types. They’re especially useful in defining types like Option and Result for error handling.
- Pattern Matching: Rust’s match statement provides a powerful way to control flow based on data structure patterns, offering more expressive control.
This knowledge is essential when building programs that rely on state machines, complex data structures, or when handling different types of data elegantly.
Suggested Learning Path: Practice creating enums and structs, and implement pattern matching to handle different states.
6. Error Handling in Rust
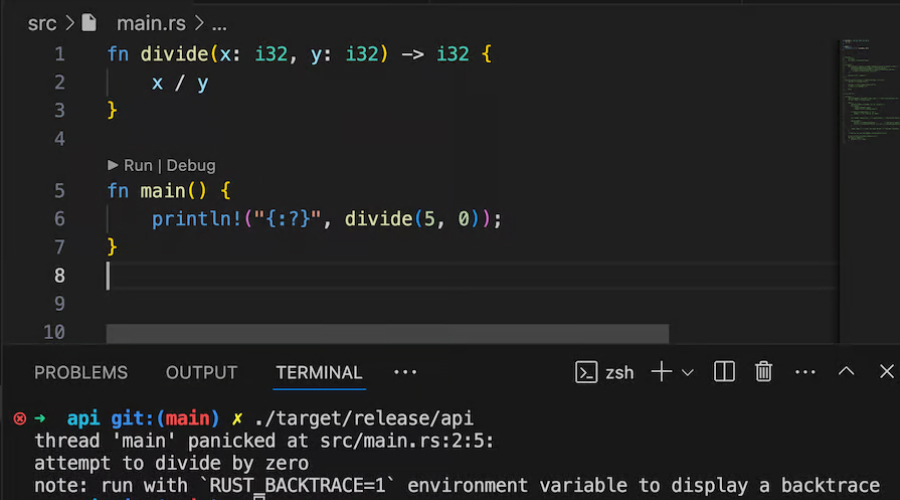
Rust handles errors using the Result and Option types, which prevent exceptions and encourage handling all possible error cases.
- Result and Option Types: Result for functions that can fail and Option for cases where values might be absent.
- Error Propagation: Use the ? operator for cleaner error handling and to propagate errors in a concise way.
- Creating Custom Errors: Create enums that represent errors specific to your application, making your code more maintainable.
Suggested Learning Path: Work on small projects, practicing error handling with Result and Option to develop resilient Rust applications.
7. Using Traits and Generics
- Traits: Rust’s version of interfaces, allowing you to define shared behavior across different types.
- Generics: Enable writing flexible functions and structs that work with any data type.
Traits and generics are essential for code reusability and for working with Rust’s powerful type system.
Suggested Learning Path: Review Rust By Example on generics and traits, and implement simple examples to solidify your understanding.
8. Concurrency and Asynchronous Programming
Rust’s memory-safety guarantees make concurrency safe and reliable.
- Threads and Message Passing: Create safe and concurrent applications with threads and Rust’s std::sync module.
- Asynchronous Programming: Rust’s async model uses async and await to simplify concurrency, along with libraries like tokio and async-std.
Suggested Learning Path: Use The Async Book to learn the foundations of asynchronous Rust.
9. Unsafe Rust and FFI (Foreign Function Interface)
While Rust emphasizes memory safety, some low-level programming requires breaking Rust’s safety guarantees.
- Unsafe Code: Understanding when to use unsafe blocks, especially when interfacing with system code or optimizing critical paths.
- FFI: Rust can interface with C/C++ code, making it useful for systems programming or embedding Rust in other applications.
Suggested Learning Path: Study The Rust Book’s Unsafe Rust chapter and experiment with simple unsafe code snippets.
Also Read:
Pro Tips for Beginner Rust Developers
- Build Real-World Projects
- Testing and Benchmarking
- Explore Rust Libraries and Ecosystem
- Contribute to Open-Source Rust Projects
- Keep Up with Rust Updates
- Build a Rust Portfolio
Conclusion
The Rust Developer Roadmap is your guide to become an efficient Rust developer by 2025. You can start by learning the fundamentals, installing your IDE, and getting hands-on experience with actual projects, which will help you to become an expert in Rust in no time. First, the most significant factors that need to be discussed are ownership, error handling, and async programming in further detail.
Connect with the Rust community, take part in real projects and keep self-development at hand. Rust’s high performance safety and growing proliferation makes it a very good language to learn now to have a promising future in technology.